print("와챠의 개발 기록장")
[React]#2-2 Inflearn: 만들면서 배우는 리액트: 기초/event/useState/상태 끌어올리기/네이밍 컨벤션 본문
[React]#2-2 Inflearn: 만들면서 배우는 리액트: 기초/event/useState/상태 끌어올리기/네이밍 컨벤션
minWachya 2023. 1. 19. 20:20목차
- 스타일링(css 클래스, 인라인 스타일링)
- Emotion
- TailWind Css
- 핸들러 네이밍 컨벤션
- Event: form 제출 시 리프레시 막기
- useState로 상태 만들기
- 상태 끌어올리기(lifting state up)
1. 스타일링
두가지 방법이 있다.
- CSS 클래스명을 넘기는 것
- 인라인으로 스타일링하는 것이다.
기존 css코드는 아래와 같다.
.favorites img {
width: 150px;
}
CSS 클래스:
리액트 태크랑 html 태그랑 조금 다르다.
class => className
<div className="danger">위험</div>
인라인 스타일링:
<div style={{ color: 'red' }}>위험</div>
다른 예시로는
<img src={img} style={{ width: "150px", border: '1px solid red' }} />
- 중괄호 2개!
- 오브젝트 사용해서 써주기!
- key, value에서 value는 string으로 써주기!
- 요소 여러개면 쉼표로 구분!
최근 리액트 스타일링 트렌드
1-1. Emotion
Emotion – Introduction
(Edit code to see changes)
emotion.sh
스타일 prop을 넘기는 게 아닌, css prop을 string으로 넘김
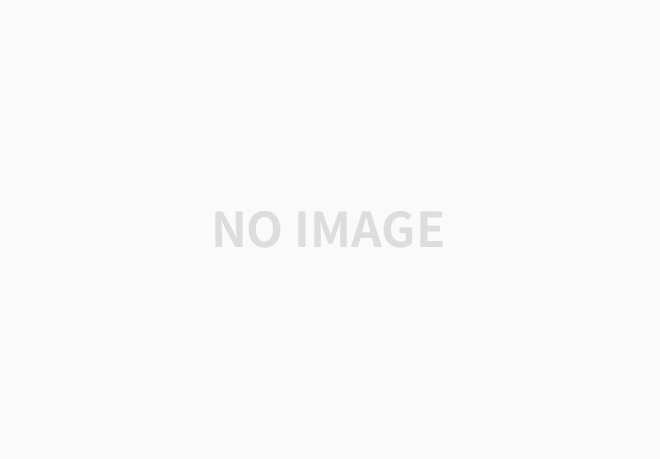
또 스타일드 컴포넌트라고 css를 가진 컴포넌트도 사용할 수 있음
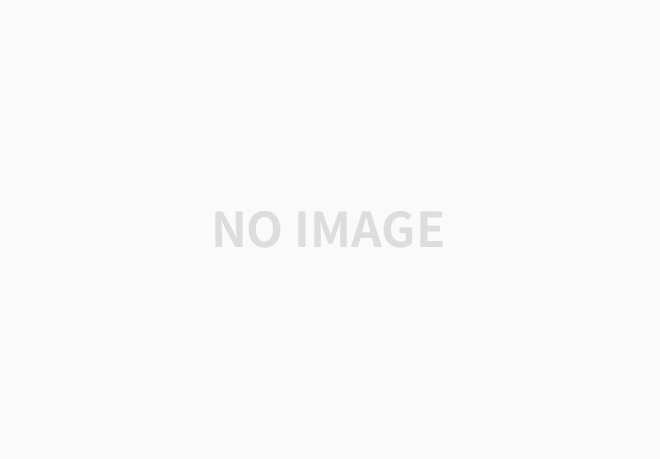
1-2. TailWind CSS
Tailwind CSS - Rapidly build modern websites without ever leaving your HTML.
Documentation for the Tailwind CSS framework.
tailwindcss.com
css, prop넘기는 게 아니라 className을 미리 정해놔
클래스 이름만 알면 빠르게 스타일링 할 수 있음.
2. 핸들러 네이밍 컨벤션
리액트는 카멜케이스를 따른다.(onclick -> onClick )
리액트에서 이벤트 핸들러 네이밍 관례는 아래와 같다.
onClick의 핸들러: handle + A + Click
onMouseOver의 핸들러: handel + A + MouseOver
<input
type="text"
name="name"
value={value}
placeholder="영어 대사를 입력해주세요"
onChange={handelInputChange}
/>
+ 함수를 prop으로 넘길 때 컨벤션은 앞에 on 을 붙이는 것이다.
ex) onHandelHeartClick
<MainCard img={mainCatImage} onHandleHeartClick={handleHeartClick} />
const MainCard = ({ img, onHandleHeartClick }) => {
return (
<div className="main-card">
<img
src={img}
alt="고양이"
width="400"
/>
<button onClick={onHandleHeartClick}>🤍</button>
</div>
);
};
3. Event: form 제출 시 리프레시 막기
event.preventDefault();
이렇게 사용함
const Form = () => {
function handelFormSubmit(event) {
event.preventDefault();
console.log("폼 전송됨");
}
return (
<form onSubmit={handelFormSubmit}>
<input type="text" name="name" placeholder="영어 대사를 입력해주세요" />
<button type="submit">생성</button>
</form>
);
};
4. useState로 상태 만들기
상태는 리액트의 useState로 만들고 바꿀 수 있음.
아래 코드와 같이 상태를 생성하고 바꿀 수 있다.
이때 상태는 컴포넌트 안에서 만들 수 있다.
const [value, setValue] = React.useState(초기값);
생성 버튼 클릭 시 title의 "n번째 고양이 가라사대"의 n<< +1해주기를 구현해보자.
const Form = () => {
const counterState = React.useState(1); // 초기값은 1
// counterState는 2개로 니뉨
const counter = counterState[0]; // counterState의 0번째 인자
const setCounter = counterState[1]; // counterState의 1번째 인자
console.log("카운터", counter);
function handelFormSubmit(event) {
event.preventDefault();
setCounter(counter + 1); // +1 해주기
}
return (
<form onSubmit={handelFormSubmit}>
<input type="text" name="name" placeholder="영어 대사를 입력해주세요" />
<button type="submit">생성</button>
</form>
);
};
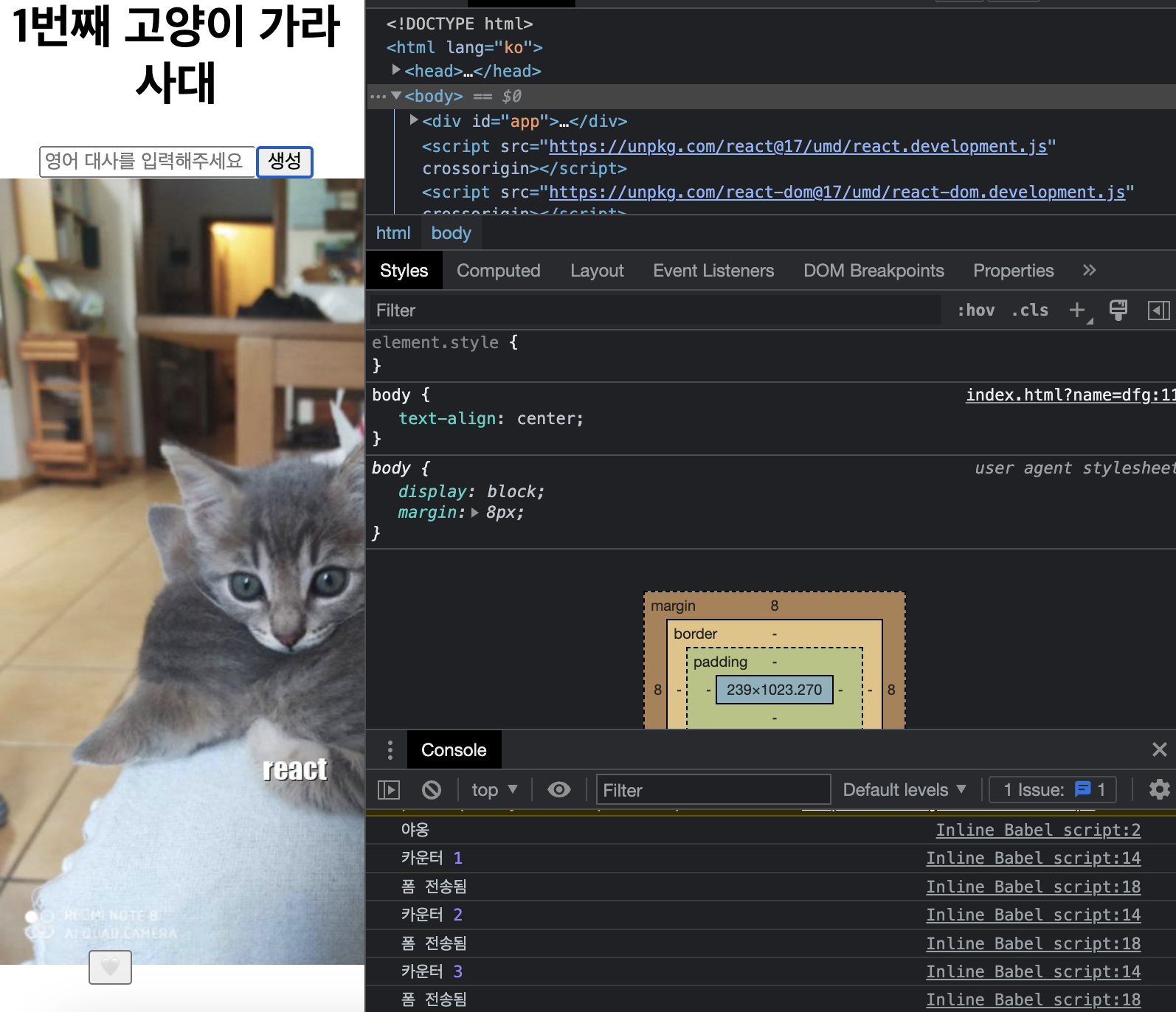
+
const counterState = React.useState(1); // 초기값은 1
// counterState는 2개로 니뉨
const counter = counterState[0]; // counterState의 0번째 인자
const setCounter = counterState[1]; // counterState의 1번째 인자
const [counter, setCounter] = React.useState(1); // 초기값은 1
이렇게 간단히 쓸 수 있음
5. 상태 끌어올리기(lifting state up)
자식 컴포넌트 안에서 쓰이던 State를
부모 컴포넌트에서 같이 쓰고 싶을 때
상태 끌어올리기 (lifting state up) 사용!!
위 코드(3번)를 보면 Form 안에 counter를 만들었기 때문에 이를 Title에 넣을 수 없음.
Title에서도 이 counter를 사용해주기 위해 Form에서 만든 State를 부모 컴포넌트(App)으로 올려준다.
const App = () => {
// 여기로 끌어올리기
const [counter, setCounter] = React.useState(1); // 초기값은 1
console.log("카운터", counter);
function handelFormSubmit(event) {
event.preventDefault();
setCounter(counter + 1);
}
return (
/* 그러면 요로코롬 Title에서도 count사용 가능 */
<div>
<Title>{counter}번째 고양이 가라사대</Title>
<Form handelFormSubmit={handelFormSubmit} />
<MainCard img="https://cataas.com/cat/HSENVDU4ZMqy7KQ0/says/react" />
<Favorites />
</div>
);
};
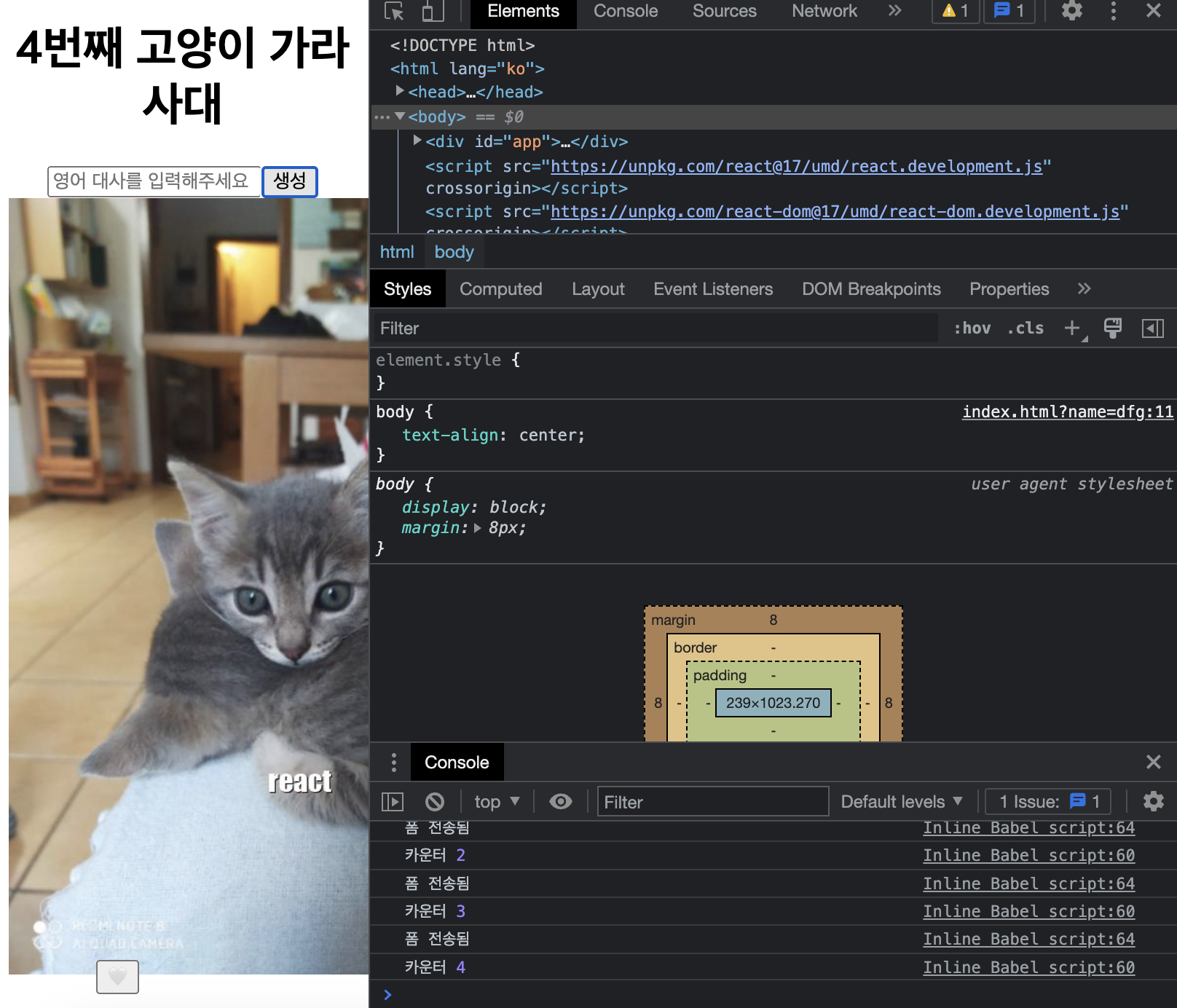